Describe how to teach high school students to program a console app using C#
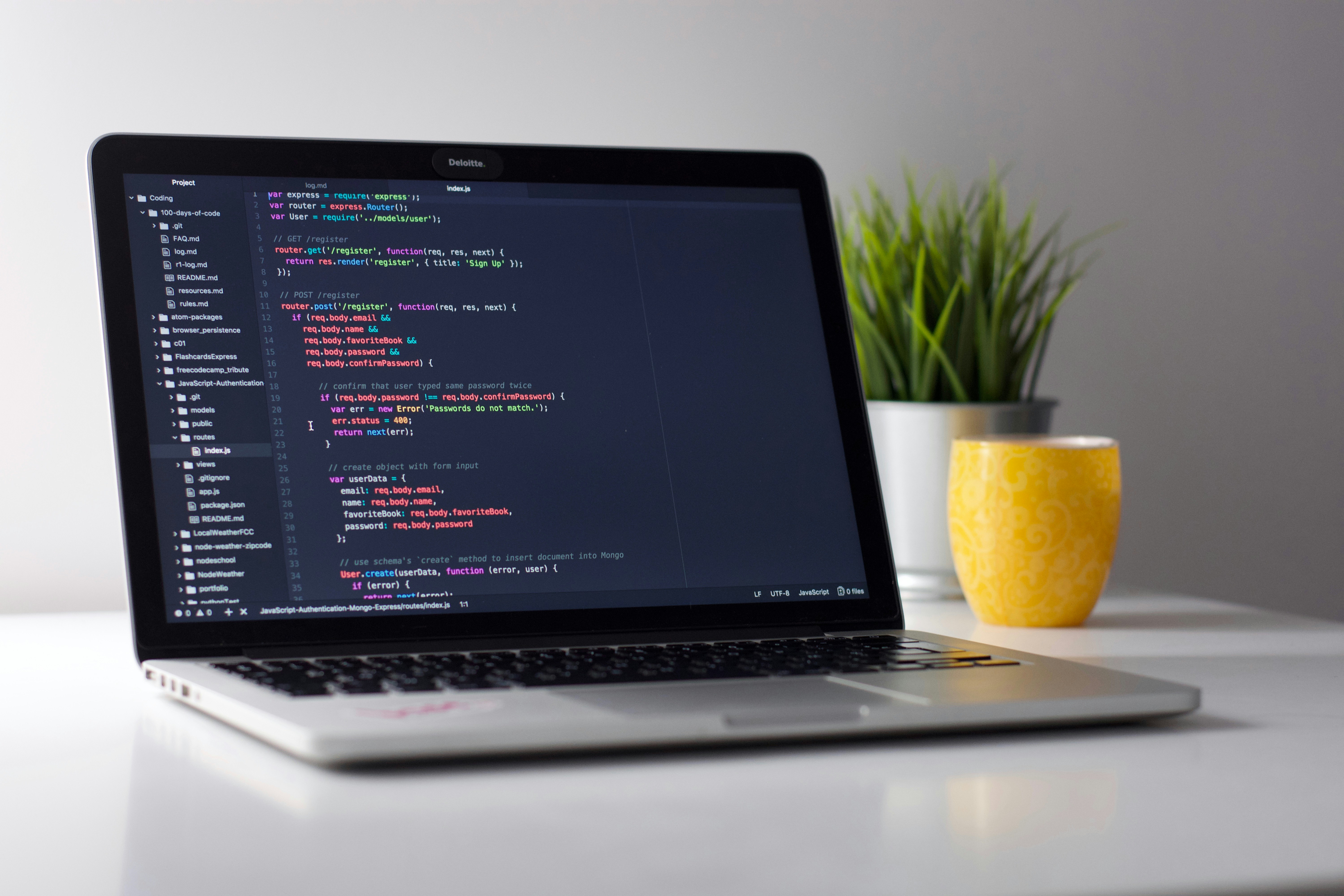
Teaching high school students to program a console app using C# can be a rewarding and valuable experience for both the teacher and the students. Here are some steps you can follow to effectively teach this topic:
- Start with the basics: Before diving into coding, make sure students have a solid understanding of the fundamentals of programming such as variables, data types, loops, and conditional statements. You can use simple examples and exercises to reinforce these concepts.
- Introduce C#: Explain the basics of C# programming language to students including syntax, keywords, and basic concepts. Provide examples and explanations to help students understand how C# works.
- Set up the development environment: Make sure students have access to a development environment where they can write and test their code. Visual Studio is a popular choice for C# programming and is user-friendly for students.
- Teach console app programming: Begin by teaching students how to create a console application in C# using Visual Studio. Show them how to create a new project, write code in the Main method, and run the application to see the output in the console window.
- Demonstrate input and output: Teach students how to read user input from the console and display output using the Console.ReadLine() and Console.WriteLine() methods. Use simple examples to demonstrate how to get user input and display messages on the console.
By following these steps and providing a supportive learning environment, you can effectively teach high school students to program a console app using C# and help them develop valuable skills in programming and problem-solving.
Describe how to teach high school students to use HTML and CSS
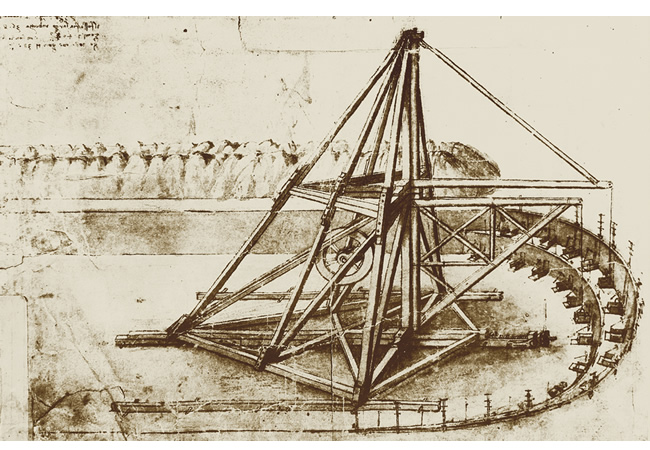
- Start with the basics: Begin the lesson by explaining what HTML and CSS are and how they are used in web development. Provide examples of websites that utilize HTML and CSS to show students the real-world applications of these languages.
- Hands-on exercises: Encourage students to practice coding in HTML and CSS by providing them with hands-on exercises. Start with simple tasks such as creating a basic webpage with text and images, and gradually increase the complexity of the exercises as students become more comfortable with the languages.
- Collaborative projects: Assign students to work on collaborative projects where they can apply their HTML and CSS skills to create a complete website. This will not only help them practice their coding skills but also improve their communication and teamwork abilities.
- Provide resources: Offer students access to online resources and tutorials where they can continue to learn about HTML and CSS outside of the classroom. Encourage them to explore different websites and experiment with new coding techniques to enhance their skills.
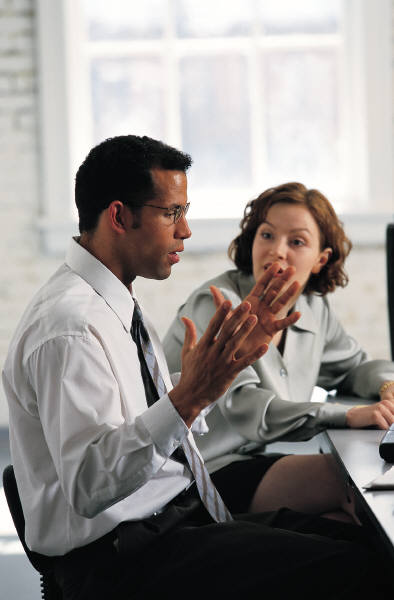
Explain in depth how to write JavaScript for a web page that acts like a calculator
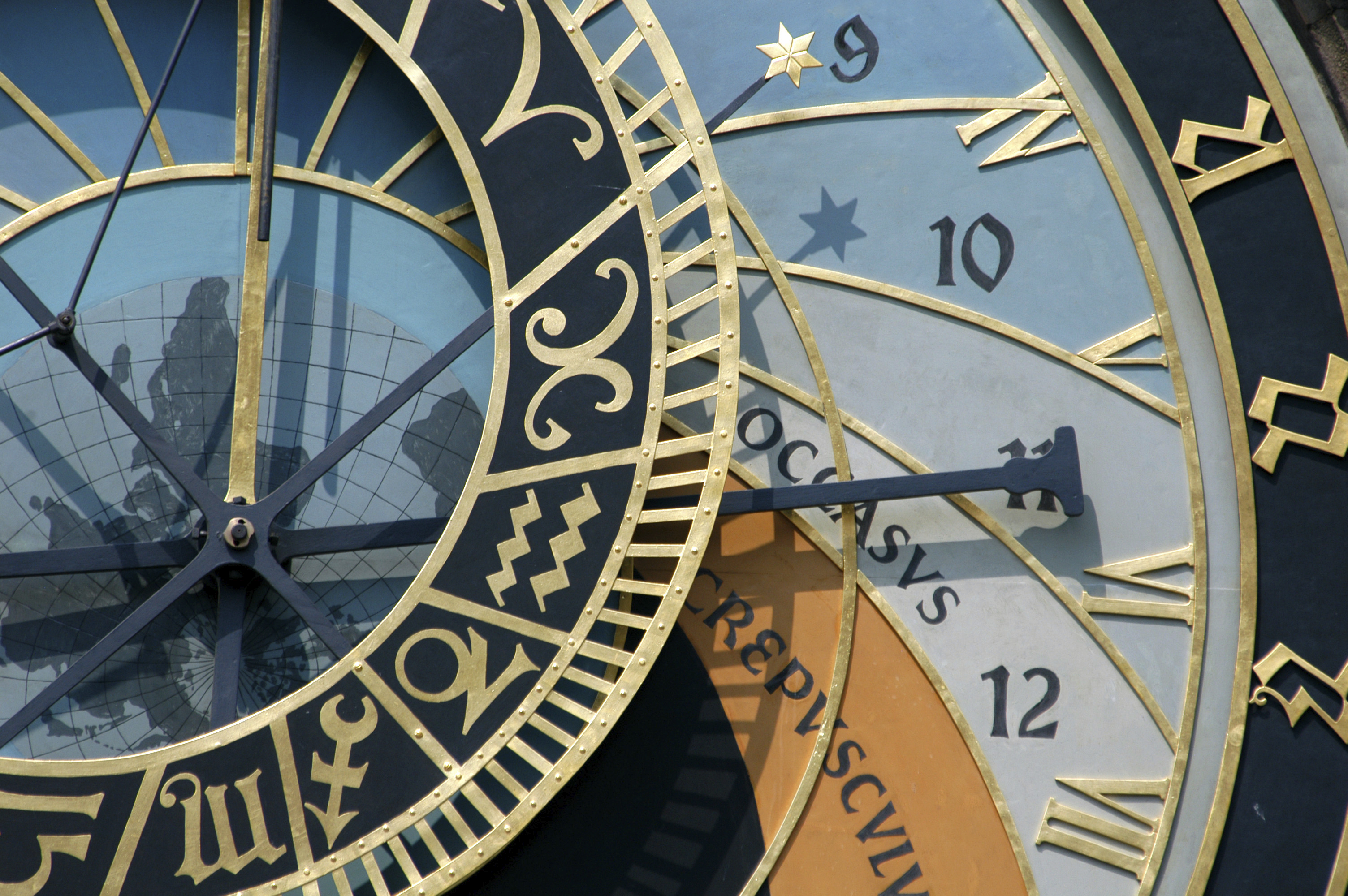
To create a web page that acts like a calculator using JavaScript, you can follow these steps:
- Create a basic HTML structure: Start by creating a basic HTML structure for your calculator page. Create input elements for displaying the user input and result, and create button elements for the numbers and operators.
-
Write JavaScript functions for the calculator operations: Create JavaScript functions for the different operations of the calculator.
let input = document.getElementById("input"); let result = document.getElementById("result"); let expression = ""; function appendNumber(num) { expression += num.toString(); input.value = expression; } function appendOperator(operator) { expression += operator; input.value = expression; } function clearInput() { expression = ""; input.value = ""; result.value = ""; } function calculate() { try { result.value = eval(expression); expression = ""; input.value = ""; } catch (error) { result.value = "Error"; expression = ""; } }
- Link JavaScript file to HTML: Save the JavaScript code in a separate file (e.g., calculator.js) and link it to your HTML file.
- Test the calculator: Open the HTML file in your web browser and test your calculator by entering numbers and operators and clicking the "=" button to get the result.
Your calculator web page should now be functional, allowing users to perform basic arithmetic operations. Feel free to customize the design and functionality of the calculator further based on your requirements.